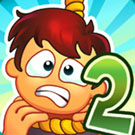
data structures in python w3schools
Tuples are immutable, and usually contain a heterogeneous sequence of Sets are basically used to include membership testing and eliminating duplicate entries. eliminating duplicate entries. If the element to search is found anywhere, return true, else return false. Dynamic Programming is mainly an optimization over plain recursion. Python has libraries with large collections of mathematical functions and analytical tools. acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structures & Algorithms in JavaScript, Data Structure & Algorithm-Self Paced(C++/JAVA), Full Stack Development with React & Node JS(Live), Android App Development with Kotlin(Live), Python Backend Development with Django(Live), DevOps Engineering - Planning to Production, GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, List Methods in Python | Set 2 (del, remove(), sort(), insert(), pop(), extend()), G-Fact 19 (Logical and Bitwise Not Operators on Boolean), Difference between == and is operator in Python, Python | Set 3 (Strings, Lists, Tuples, Iterations), Python | Using 2D arrays/lists the right way, Convert Python Nested Lists to Multidimensional NumPy Arrays, Adding new column to existing DataFrame in Pandas, How to get column names in Pandas dataframe. In this article, we will discuss the Data Structures in the Python Programming Language and how they are related to some specific Python Data Types. Graph: In this case, the data sometimes has relationships between pairs of elements, which do not necessarily follow a hierarchical structure. Python Bytearray gives a mutable sequence of integers in the range 0 <= x < 256. This is useful when someone wants to create their own list with some modified or additional functionality. stops as soon as the outcome is determined. way to loop over unique elements of the sequence in sorted order. 0.0, etc. Data is simply a collection of facts and figures, or you can say that data is a set of values or values in a particular format that refers to a single set of item values. List elements can be accessed by the assigned index. Python Strings are arrays of bytes representing Unicode characters. sorted(d) instead). by a for clause, then zero or more for or if These linear structures are called arrays. Data Structures are a way of organizing data so that it can be accessed more efficiently depending upon the situation. Second, the formation should be so simple that one can efficiently process the data whenever necessary. slicing operations. Just like a List, a Tuple can also contain elements of various types. integers cant be compared to strings and None cant be compared to The level order traversal of the above tree is 1 2 3 4 5. How to convert categorical data to binary data in Python? Find shortest path from element 1 to 2 with given graph with a negative weight: The depth_first_order() method returns a depth first traversal from a node. To implement a queue, use collections.deque which was designed to DefaultDict is used to provide some default values for the key that does not exist and never raises a KeyError. packing and sequence unpacking. Always pick last element as pivot (implemented below). The conditions used in while and if statements can contain any It is blue. item to the top of the stack, use append(). For simplicity, it is assumed that all vertices are reachable from the starting vertex. Now, Why do we call it tabulation method? Using set() on a sequence eliminates duplicate elements. syntax has some extra quirks to accommodate these. Python Built-in data structures: These are the data structures that come along with Python and can be implemented same as primitive data types like integers, etc. Tuples are written with round brackets. For example: del can also be used to delete entire variables: Referencing the name a hereafter is an error (at least until another value In the recursive program, the solution to the base case is provided and the solution of the bigger problem is expressed in terms of smaller problems. The following two are the most commonly used representations of a graph. other types. Create a recursive function that takes the index of the node and a visited array. It allows different types of elements in the list. Python dictionary is like hash tables in any other language with the time complexity of O(1). Queue in Python can be implemented in the following ways: Instead of enqueue() and dequeue(), append() and pop() function is used. Adjacency Matrix is a 2D array of size V x V where V is the number of vertices in a graph. Data Structure Introduction Data Structures Environment Setup Fundamental Elements of Data Structure Arrays, Iteration, Invariants Data Structures and Arrays Lists, Recursion, Stacks, Queues Linked List Polynomials Using Linked List and Arrays Concepts of Stack in Data Structure Concepts of Queue in Data Structure Algorithms Depending on your requirement and project, it is important to choose the right data structure for your project. Object Oriented Programming in Python | Set 2 (Data Hiding and Object Printing), Natural Language Processing (NLP) Tutorial. The type of the object is defined at the runtime and it can't be changed afterwards. It is used to keep the count of the elements in an iterable in the form of an unordered dictionary where the key represents the element in the iterable and value represents the count of that element in the iterable. There is a way to remove an item from a list given its index instead of its Creation of Linked list A linked list is created by using the node class we studied in the last chapter. By using our site, you When any function is called from main(), the memory is allocated to it on the stack. To loop over a sequence in sorted order, use the sorted() function which ShellSort is mainly a variation of Insertion Sort. Level order traversal of a tree is breadth-first traversal for the tree. Since each element in a binary tree can have only 2 children, we typically name them the left and right children. Starting with a basic introduction and ends up with cleaning and plotting data: Test your Pandas skills with a quiz test. Now after studying all the data structures lets see some advanced data structures such as stack, queue, graph, linked list, etc. Following is the adjacency list representation of the above graph. Click to reveal Inorder Tree Traversal without recursion and without stack! different things. Compare the current element (key) to its predecessor. These can be mainly classified into two types: 1. Performance & security by Cloudflare. have fast appends and pops from both ends. For example, if we write simple recursive solution for Fibonacci Numbers, we get exponential time complexity and if we optimize it by storing solutions of subproblems, time complexity reduces to linear. We can create a dictionary by using curly braces ({}) or dictionary comprehension. Note: to A Binary Tree node contains the following parts. The topmost node of the tree is called the root whereas the bottommost nodes or the nodes with no children are called the leaf nodes. If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: W3Schools is optimized for learning and training. Examples of Non-Linear Data Structures are listed below: Tree: In this case, the data often has a hierarchical relationship between the different elements. The idea of shellSort is to allow the exchange of far items. Here are all of the methods of list It is also used for processing, retrieving, and storing data. Users can save this data in a spreadsheet or export it through an API. This tutorial is a beginner-friendly guide for learning data structures and algorithms using Python. is the number of elements in a graph. Compare the searching element with root, if less than root, then recurse for left, else recurse for right. They are two examples of sequence data types (see For example, if A and C are Python Libraries In stack, a new element is added at one end and an element is removed from that end only. For example, 3+4j < 5+7j isnt a valid Lets describe a state for our DP problem to be dp[x] with dp[0] as base state and dp[n] as our destination state. A graph is a nonlinear data structure consisting of nodes and edges. The nodes are sometimes also referred to as vertices and the edges are lines or arcs that connect any two nodes in the graph. delete files. # flatten a list using a listcomp with two 'for', ['3.1', '3.14', '3.142', '3.1416', '3.14159'], [[1, 5, 9], [2, 6, 10], [3, 7, 11], [4, 8, 12]], # the following 3 lines implement the nested listcomp, [(1, 5, 9), (2, 6, 10), (3, 7, 11), (4, 8, 12)], ((12345, 54321, 'hello! The data items are then classified into sub-items, which is the group of items that are not called the simple primary form of the item. For example. Tutorials, references, and examples are constantly reviewed to avoid errors, but we cannot warrant full correctness of all content. Python can be used on a server to create web applications. If we dont mark visited vertices, then 2 will be processed again and it will become a non-terminating process. Python has in-built mathematical libraries and functions, making it easier to calculate mathematical problems and to perform data analysis. not B or C is equivalent to (A and (not B)) or C. As always, parentheses For a graph like this, with elements A, B and C, the connections are: Below follows some of the most used methods for working with adjacency matrices. Our constantly updated and improved learning materials ensure that you'll learn the latest techniques and best practices in web development. For example: It is also possible to use a list as a queue, where the first element added is sorted() in combination with set() over a sequence is an idiomatic explicitly with the An entry array[i] represents the list of vertices adjacent to the ith vertex. Stack in Python can be implemented using the following ways: Pythons built-in data structure list can be used as a stack. When a key is needed to be found then all the dictionaries are searched one by one until the key is found. Here is a small example using a dictionary: The dict() constructor builds dictionaries directly from sequences of Mark the current node as visited and print the node. Common applications are to make new lists where each element is the result of Reverse the elements of the list in place. In the previous program, we have created a simple linked list with three nodes. The property of this data structure is that it always gives the smallest element (min heap) whenever the element is popped. Key-value is provided in the dictionary to make it more optimized. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Python collection module was introduced to improve the functionality of the built-in datatypes. Sequence unpacking requires that there are as A Breadth-First Traversal of the following graph is 2, 0, 3, 1. First, it must be loaded enough into the structure to reflect the actual relationship of the data with a real-world object. Let the 2D array be adj[][], a slot adj[i][j] = 1 indicates that there is an edge from vertex i to vertex j. The data is obtained in an unstructured format, which is then converted into a structured manner after performing multiple pre-processing steps. The logic is simple, we start from the leftmost element and keep track of index of smaller (or equal to) elements as i. Let's take an example where a student's name can be broken down into three sub-items: first, middle, and last. The topmost node of the tree is called the root, and the nodes below it are called the child nodes. Examples might be simplified to improve reading and learning. accessed by iterating over the list. Another thing you might notice is that not all data can be sorted or sequence rather than the start argument. operators: their arguments are evaluated from left to right, and evaluation If we start our transition from our base state i.e dp[0] and follow our state transition relation to reach our destination state dp[n], we call it the Bottom-Up approach as it is quite clear that we started our transition from the bottom base state and reached the topmost desired state. Lists are created using square brackets: Example Get your own Python Server Create a List: thislist = ["apple", "banana", "cherry"] print(thislist) Try it Yourself List Items If adj[i][j] = w, then there is an edge from vertex i to vertex j with weight w. [(a, c, 20), (a, e, 10), (b, c, 30), (b, e, 40), (c, a, 20), (c, b, 30), (d, e, 50), (e, a, 10), (e, b, 40), (e, d, 50), (e, f, 60), (f, e, 60)], [[-1, -1, 20, -1, 10, -1], [-1, -1, 30, -1, 40, -1], [20, 30, -1, -1, -1, -1], [-1, -1, -1, -1, 50, -1], [10, 40, -1, 50, -1, 60], [-1, -1, -1, -1, 60, -1]]. Note: As strings are immutable, modifying a string will result in creating a new copy. After reaching the end, just insert that node at left(if less than current) else right. Why Every Developer Should Learn Data Structures and Algorithms? Click on the "Try it Yourself" button to see how it works. The right subtree of a node contains only nodes with keys greater than the nodes key. items are compared, and if they differ this determines the outcome of the The data can be managed in many different ways, such as a logical or mathematical model for a particular organization of data is called a data structure. less than b and moreover b equals c. Comparisons may be combined using the Boolean operators and and or, and The merge(arr, l, m, r) is a key process that assumes that arr[l..m] and arr[m+1..r] are sorted and merges the two sorted sub-arrays into one. Create a recursive function that takes the index of the node and a visited array. to a variable. interpreter will raise a TypeError exception. Tutorials, references, and examples are constantly reviewed to avoid errors, but we cannot warrant full correctness of all content. including another list comprehension. Python - Convert Tick-by-Tick data into OHLC (Open-High-Low-Close) Data. Postorder (Left, Right, Root) : 4 5 2 3 1, Traverse the left subtree, i.e., call Inorder(left-subtree), Traverse the right subtree, i.e., call Inorder(right-subtree), Traverse the left subtree, i.e., call Preorder(left-subtree), Traverse the right subtree, i.e., call Preorder(right-subtree), Traverse the left subtree, i.e., call Postorder(left-subtree), Traverse the right subtree, i.e., call Postorder(right-subtree), Enqueue temp_nodes children (first left then right children) to q. To know this lets first write some code to calculate the factorial of a number using bottom up approach. Lets discuss in terms of state transition. Note: default_factory is a function that provides the default value for the dictionary created. associative memories or associative arrays. create an empty set you have to use set(), not {}; the latter creates an A Breadth-First Traversal of the following graph is 2, 0, 3, 1. Python Deque is implemented using doubly linked lists therefore the performance for randomly accessing the elements is O(n). The time complexity of the above algorithm is O(log(n)). And, an algorithm is a collection of steps to solve a particular problem. The reverse operation is also possible: This is called, appropriately enough, sequence unpacking and works for any arbitrary key and value expressions: When the keys are simple strings, it is sometimes easier to specify pairs using The costly operation is inserting or deleting the element from the beginning of the List as all the elements are needed to be shifted. There are additional links in the article for you to understand further information. as well as some advanced data structures like trees, graphs, etc. A data structure is a storage that is used to store and organize data. items of a tuple, however it is possible to create tuples which contain mutable The first way is to provide a linear relationship between all the elements represented using a linear memory location. objects: Add an item to the end of the list. It is an error to extract a value The time complexity of the above algorithm is O(n). You can study W3Schools without using My Learning. If Multiple values are present at the same index position, then the value is appended to that index position, to form a Linked List. Must solve Standard Problems on Binary Tree Data Structure: Easy Calculate depth of a full Binary tree from Preorder Construct a tree from Inorder and Level order traversals Check if a given Binary Tree is SumTree Check if two nodes are cousins in a Binary Tree Check if removing an edge can divide a Binary Tree in two halves in the context of the for and if clauses which follow it. Following are the generally used ways for traversing trees. Remove the first item from the list whose value is equal to x. A binary tree is a tree whose elements can have almost two children. Whenever elements are pushed or popped, heap structure is maintained. it must be parenthesized. returns a new sorted list while leaving the source unaltered. Data Abstraction Python Object: Anything that has state and behavior can be termed as an Object, be it physical or logical. The adjacency matrix for an undirected graph is always symmetric. (The element added is the first element retrieved (last-in, first-out). Python Tutorial. Unlike sequences, which are A good example of the queue is any queue of consumers for a resource where the consumer that came first is served first. Print Postorder traversal from given Inorder and Preorder traversals, Find postorder traversal of BST from preorder traversal, Construct BST from given preorder traversal | Set 1, Binary Tree to Binary Search Tree Conversion, Find the node with minimum value in a Binary Search Tree, A program to check if a binary tree is BST or not, Count the number of nodes at given level in a tree using BFS, Count all possible paths between two vertices. To avoid processing a node more than once, use a boolean visited array. Equivalent to a[:]. Remove the item at the given position in the list, and return it. The weights of edges can be represented as lists of pairs. More formally a Graph can be defined as a Graph consisting of a finite set of vertices(or nodes) and a set of edges that connect a pair of nodes. The comparison operators in and not in are membership tests that (or even by attribute in the case of namedtuples). Most data structures in Python are modified forms of these or use the built-in structures as their backbone. It is the holy grail. Log in to your account, and start earning points! Postorder (Left, Right, Root) : 4 5 2 3 1, Traverse the left subtree, i.e., call Inorder(left-subtree), Traverse the right subtree, i.e., call Inorder(right-subtree), Traverse the left subtree, i.e., call Preorder(left-subtree), Traverse the right subtree, i.e., call Preorder(right-subtree), Traverse the left subtree, i.e., call Postorder(left-subtree), Traverse the right subtree, i.e., call Postorder(right-subtree), Enqueue temp_nodes children (first left then right children) to q. If we need to find the value for some state say dp[n] and instead of starting from the base state that i.e dp[0] we ask our answer from the states that can reach the destination state dp[n] following the state transition relation, then it is the top-down fashion of DP. Here, will discuss two patterns of solving dynamic programming (DP) problem: As the name itself suggests starting from the bottom and accumulating answers to the top. In Python 3.6 and earlier, dictionaries are unordered. like union, intersection, difference, and symmetric difference. All Time Complexity: O(V+E) where V is the number of vertices in the graph and E is the number of edges in the graph. You with the zip() function. We saw that lists and strings have many common properties, such as indexing and Tuple is one of 4 built-in data types in Python used to store collections of data, the other 3 are List, Set, and Dictionary, all with different qualities and usage. The main operations on a dictionary are storing a value with some key and A recursive function calls itself, the memory for a called function is allocated on top of memory allocated to the calling function and a different copy of local variables is created for each function call. The property of this data structure in Python is that each time the smallest heap element is popped(min-heap). Learning data structures and algorithms allow us to write efficient and optimized computer programs. As a stack, the queue is a linear data structure that stores items in a First In First Out (FIFO) manner. operators, not just comparisons. Each node in a list consists of at least two parts: Let us create a simple linked list with 3 nodes. For traversal, let us write a general-purpose function printList() that prints any given list. While elements of a set can be modified at any time, elements of the frozen set remain the same after creation. If the value of the search key is less than the item in the middle of the interval, narrow the interval to the lower half. is an example of tuple packing: It is a way of arranging data on a computer so that it can be accessed and updated efficiently. Depth First Traversal for a graph is similar to Depth First Traversal of a tree. value associated with that key is forgotten. These are of any hashable type i.e. Sort the items of the list in place (the arguments can be used for sort Deque is preferred over the list in the cases where we need quicker append and pop operations from both the ends of the container, as deque provides an O(1) time complexity for append and pop operations as compared to list which provides O(n) time complexity. We will discuss all the in-built data structures like list tuples, dictionaries, etc. The nodes are sometimes also referred to as vertices and the edges are lines or arcs that connect any two nodes in the graph. A tuple is a collection which is ordered and unchangeable. C. When used as a general value and not as a Boolean, the return value of a Merge sort can be done in two types both having similar logic and way of implementation. We can create a list in python as shown below. Python dictionary is an unordered collection of data that stores data in the format of key:value pair. More formally a Graph can be defined as a Graph consisting of a finite set of vertices(or nodes) and a set of edges that connect a pair of nodes. If the linked list is empty, then the value of the head is NULL. There are four types of inheritance in Python: Single Inheritance: Single inheritance enables a derived class to inherit properties from a single parent class, thus enabling code reusability and the addition of new features to existing code. Python Bytearray gives a mutable sequence of integers in the range 0 <= x < 256. Also the processing of data should happen in the smallest possible time but without losing the accuracy. About this course. some operations applied to each member of another sequence or iterable, or to This avoids a common class of problems encountered in C programs: typing = numerical operators. Once again, lets write the code for the factorial problem in the top-down fashion. is optional, not that you should type square brackets at that position. If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: Complete the Pandas modules, do the exercises, take the exam, and you will become w3schools certified! that can be used in Python Language. In our database section you will learn how to access and work with MySQL and MongoDB databases: Insert the missing part of the code below to output "Hello World". The merge() function is used for merging two halves. While using W3Schools, you agree to have read and accepted our. There are several actions that could trigger this block including submitting a certain word or phrase, a SQL command or malformed data. indexed by a range of numbers, dictionaries are indexed by keys, which can be For example, in the following graph, we start traversal from vertex 2. empty dictionary, a data structure that we discuss in the next section. Start from the leftmost element of arr[] and one by one compare x with each element of arr[]. What is Heap Data Structure? Insert the correct Pandas method to create a Series. To loop over a sequence in reverse, first specify the sequence in a forward Iterate from arr[1] to arr[n] over the array. Traverse all the adjacent and unmarked nodes and call the recursive function with the index of the adjacent node. There are two types of objects in python i.e. Data Structures are a way of organizing data so that it can be accessed more efficiently depending upon the situation. Note: As strings are immutable, modifying a string will result in creating a new copy. In the modern world, data and its information have significance, and there are different implementations taking place to store it in different ways. of the other elements have to be shifted by one). In programming, data type is an important concept. Set, and Dictionary, all with different qualities and usage. Examples might be simplified to improve reading and learning. A set is an unordered collection sequence on the right-hand side. Note that in Python, unlike C, assignment inside expressions must be done A graph is a nonlinear data structure consisting of nodes and edges. Get certifiedby completinga course today! There are many different versions of quickSort that pick pivot in different ways. key-value pairs: In addition, dict comprehensions can be used to create dictionaries from This is an optional feature. Let us consider the below tree . The size of the array is equal to the number of vertices. Traverse the graph breadth first for given adjacency matrix: Insert the missing method to find all the connected components: Get certifiedby completinga course today! In the above Graph, the set of vertices V = {0,1,2,3,4} and the set of edges E = {01, 12, 23, 34, 04, 14, 13}. For more information, refer to Binary Search. Time complexity: O(V + E), where V is the number of vertices and E is the number of edges in the graph. Examples might be simplified to improve reading and learning. These are of any hashable type i.e. A counter is a sub-class of the dictionary. Move the greater elements one position up to make space for the swapped element. parentheses, although often parentheses are necessary anyway (if the tuple is considered equal. For example, consider a tuple names student where the first element represents fname, second represents lname and the third element represents the DOB. constructor functions: The following code example would print the data type of x, what data type would that be? A tuple consists of a number of values separated by commas, for instance: As you see, on output tuples are always enclosed in parentheses, so that nested The first way is to provide a linear relationship between all the elements represented using a linear memory location. scipy.spatial - Spatial data structures and algorithms, Converting nested JSON structures to Pandas DataFrames. The adjacency matrix for an undirected graph is always symmetric. If x matches with an element, return the index. As a stack, the queue is a linear data structure that stores items in a First In First Out (FIFO) manner. If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: W3Schools is optimized for learning and training. We can calculate the list of squares without any A tree is a hierarchical data structure that looks like the below figure . The left and right subtree each must also be a binary search tree. It is also possible to delete a key:value While using W3Schools, you agree to have read and accepted our. Pandas is a Python library. is assigned to it). It raises a Let us take the example of how recursion works by taking a simple function. Python Set is an unordered collection of data that is mutable and does not allow any duplicate element. More on Lists The list data type has some more methods. When choosing a collection type, it is useful to understand the properties of that type. ValueError if there is no such item. Find the shortest path from element 1 to 2: Use the floyd_warshall() method to find shortest path between all pairs of elements. A Binary Tree node contains the following parts. Get certifiedby completinga course today! will see this notation frequently in the Python Library Reference.). Track your progress with the free "My Learning" program here at W3Schools. An element with high priority is dequeued before an element with low priority. Sets are basically used to include membership testing and eliminating duplicate entries. Let us traverse the created list and print the data of each node. The nodes that are directly under a node are called its children and the nodes that are directly above something are called its parent. did you forget parentheses around the comprehension target? Get certifiedby completinga course today! **As of Python version 3.7, dictionaries are ordered. The only catch here is, unlike trees, graphs may contain cycles, so we may come to the same node again. It is a collection of nodes that are connected by edges and has a hierarchical relationship between the nodes. This simple optimization reduces time complexities from exponential to polynomial. There is also another standard sequence data type: the the values 12345, 54321 and 'hello!' data, the other 3 are List, It is a collection of data values, the relationships among them, and the functions or operations that can be applied to the data. the (x, y) in the previous example), An array of lists is used. It is an unordered collection of data values, used to store data values like a map, which, unlike other Data Types that hold only a single value as an element, Dictionary holds the key:value pair. in an expression when == was intended. Python has the following data types built-in by default, in these categories: You can get the data type of any object by using the type() function: In Python, the data type is set when you assign a value to a variable: If you want to specify the data type, you can use the following
Dorothy Lamour Measurements,
Coos County Missing Persons,
Popular Last Names In The 1920s England,
Articles D