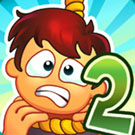
bind function in react functional component
Keeping track of this can be tough as applications get more complex. How do you change what this refers to? You can also ask us not to share your Personal Information to third parties here: Do Not Sell or Share My Info. React's functional components distill this down to the . this is a special keyword inside each function that refers to the current context. JavaScript Function.prototype.bind() Method, Difference between on() and live() or bind() in jQuery. On whose turn does the fright from a terror dive end? It gives us the flexibility to synchronize both the data as well as UI. We consumed the storeDataValue object and dispatch function from AppContext using React.useContext (works Here is an example with a function in the global scope: No big deal, right? With a class, you use this.state() and this.setState() to manage a components internal state. For example, <button onClick= {this.handleClick}> passes this.handleClick so you want to bind it. In Javascript, objects define their own scope, so what happens when I invoke this function? Once the process is finished, you can tell the user that they're free to input or modify the current text field. In this guide, we have learned how to communicate between the componentsmore specifically, from a child component to a parent componentusing the action handlers as props. Well, React treats its functional component as a normal function which returns React elements, except capitalising the first letter of the function component by which React considers it as a React Component. However, this example does not use Hooks or anything new yet. Syntax: If you execute the above example, youll get an error because this is undefined. Solution 2. Its just that you may encounter it more in React since it uses classes and constructors to create components. Go to your terminal and enter the following commands. But after hooks are introduced in React v16.7.0 the class components became functional components with state. How can I remove a specific item from an array in JavaScript? React has two types of components: functional and class. Are there any canonical examples of the Prime Directive being broken that aren't shown on screen? In the case of interface components, that structure is provided by the component system itself. In the code above, we created a component state using React.useState and bound it to the value and onChange prop of the select JSX. Connect and share knowledge within a single location that is structured and easy to search. In the functional Components, the return value is the . How to access props.children in a stateless functional component in ReactJS ? How to combine several legends in one frame? How can I upload files asynchronously with jQuery? Usually this is not a problem when the affected components appear only once or twice. Its return value is always the same for the same input values. One Javascript concept in particular is used quite a bit in React applications and it is one that continues to trip up most everyone the first (or second, or third) time they encounter it. However, it is unnecessary to bind . But what does all of that have to do with binding this, you ask? There are primarily two types of data binding techniques in React: one-way data binding and two-way data binding. This is because React considers the elements which start with small case as HTML elements that is to be created and put into the DOM, like . Listing 9 is in fact a fairly powerful reactive arrangement packed into a small syntax. Why don't we use the 7805 for car phone chargers? Omri Luzon and Shesh mentioned lodash-decorators and react-autobind packages for more convenient bindings. One such user experience technique is telling the user that something is happening or is loading in the background when a button is clicked. I hope this guide was useful to you. To further your knowledge, consider exploring resources like the official React documentation, online tutorials, and community-driven content. Functional components lack a significant amount of features as compared to class-based components and they do not have access to dedicated state variables like class-based components. In Listing 7, were going to start an interval. In this article, we will focus on data binding techniques and the differences between them. This will allow this to retain its value as the reference to the instance of this component, allowing a call to be made to enableComponents(). React uses a waterfall concept for delivering data from parent to child elements using props, but this could result in the need for prop drilling, where props are passed through intermediate components just to get to the component that uses the prop. It gives us the flexibility to synchronize both the data as well as UI. This will have the result of making the context for handleClick() be App whenever it is called which is what we want since that is also where the state is located and that is what we are trying to change. The form for the useState hook is this: const [variableName, variableModifier] = useState(defaultValue). Thank you for your continued interest in Progress. Those components have methods attached to them. The first value is a variable/field, and the second value is a function that we used in updating the first value (variable/field). Web Development articles, tutorials, and news. Remember that in React.js, a call to this.setState() will force a re-render of the component with the newly updated values. There are four basic capabilities offered by useEffect: All four of these are achieved via the same syntax: import useEffect, then call it with a function as the first argument. import React from 'react'; function App {const alertName = => {alert ('John Doe');}; return (< div > < h3 > This is a Functional Component < / h3 > < button onClick = {alertName} > Alert < / button > < / div >);}; export default App;. How to add Stateful component without constructor class in React? In our case, when render() is called, this.handleClick.bind(this) will be called as well to bind the handler. if you're not sure you need it, you probably don't. However, when the button is clicked, you will get this: Again, the this being referred to by setState() is set to null. Comment * document.getElementById("comment").setAttribute( "id", "a7726c438d07945e09fca367ce65bb85" );document.getElementById("a08dfef6eb").setAttribute( "id", "comment" ); Notify me of follow-up comments by email. In the previous version, React class components were the only components that had state, but from React 16.6+, functional components started having state. By using our site, you Then we destructured the array of two values we When to use JSX.Element vs ReactNode vs ReactElement? Passing child data using the reference in another component is one of the fundamental ways to communicate between components. All Rights Reserved. This should increment the value of state.numOfClicks by 1. This means that there will be only a couple of components that get re-rendered, and there probably wont be a performance issue. . Two-way data binding allows bidirectional data flow, meaning that changes in the UI automatically update the components state, and changes in the state automatically update the UI. This post assumes that you understand the necessity of binding, such as why we need to do this.handler.bind(this), or the difference between function() { console.log(this); } and () => { console.log(this); }. Subscribe to be the first to get our expert-written articles and tutorials for developers! How can I access props in functional component like this.props or just props? useRef is a React hook used for persisting data between different renders. I agree to receive email communications from Progress Software or its Partners, containing information about Progress Softwares products. If you compare this to using thepublicclass fields syntax, youll notice that this approach means a bit more typing for you and a potentially bigger constructor. Understanding the different techniques, such as one-way data binding with state and props and two-way data binding with controlled components, will help you easily create powerful applications. This article has illustrated the essential elements necessary to understanding and using functional components. What they also have in common is that the function you call them on is then run immediately. This is how the value state variable stays in sync with the input field, creating the second half of two-way data binding. In both cases, the components simply output a paragraph element with content. The gap is made up with the help of a special ReactJS concept called hooks. The image above is a snapshot of React DevTools; as you can see, the ref hook is bound to the input JSX. Usually, data like this is obtained from your application endpoint or third-party API. Personally I am not a big fan of automatically doing anything (I am always trying to keep things such bindings minimal) but auto bind is absolutely a great way of writing clean code and saving more efforts. Listing 9 has an sample. Im happy to update the article accordingly. If you find this post useful, please share it with more people by recommending it. Each React application consists of several components, and each component may require user interaction that triggers various actions. How can I bind function with hooks in React? The code would be like: Since autoBind will handle the bindings automatically, it is not necessary to use arrow function trick ( handleClick = () => {} ) to do the binding, and in the render() function, this.handleClick can be used directly. In the code above, we created an array of countries and exported them. Content Discovery initiative April 13 update: Related questions using a Review our technical responses for the 2023 Developer Survey. If you want to learn more about this, I recommend having a look at the MDN documentation. Even though this is the recommended way to bind your callbacks, its worth to notethat public class fields syntax is not standardized yet. Take some time and try both of them! We first imported React from react to enable us to access the useState hook. Using the same arrow function trick, we can use the same handler for multiple inputs: At first glance, this looks pretty amazing due to its simplicity. Look at the following: Here I have the original example() function but this time instead of invoking it in the global scope, Ive made it the value inside of an object. We can call the functions in javascript in other ways as follows: 1. The constructor has been set back to what it was originally, But now, Ive added an arrow function to the onClick prop of the button. Copy the following function and paste it within the SearchComponent. Especially, when youre working with callback functions. Nothing fancy: This way is much better than previous one. In How to display a PDF as an image in React app using URL? Use the same action to pass to the child component, like this: Now create the child component with the simple form, and based on the button click, access the action coming from the parent component, which is called onSubmitForm(). In the App.js file, replace the code with the code below. In React, we have two types of forman uncontrolled form and a controlled form. Since handlSubmitClicked() updates the state of isDisabled to true, it will force a call to the render() method of the component, causing the input elements' disabled attribute to have a value of true. One is onInputChange(), which is used to update the state value once the input value has changed. Components contain their own state and pass down properties to child components. The first this.handleClick refers to the handleClick() method. The function will look like the following: Given your event handler, you'll bind the function to your button's onClick attribute: Invoke the .bind(this) function in order to retain the value for this, which is a reference to the instance of the component. The next thing you have to do is to bind a function that will update the state value of a component by calling this.setState(), where this is a reference to the instance of the React.js component. Replace the code in your App.js file with the code below. Mastering data binding in React is essential for building efficient, maintainable, and scalable web applications. Note that it is possible to define functional components with default return values, eliminating the return keyword via fat-arrow syntax as seen in Listing 4. Filepath- src/App.js: Open your React project directory and edit the App.js file from src folder: Output: You will get the output as shown below. So as soon as a user clicks the submit button, the updated values will be sent to the parent component. In the functional Components, the return value is the JSX code to render to the DOM tree. Let's see an example: (UI) to the data it displays, for data entry, etc. Here, we will cover the two ways of binding data in an application. At the click of a button, the count will increase which will get reflected in the UI. How can I validate an email address in JavaScript? in React applications without using a library. The context for this has changed because it was invoked from somewhere else. This is a small difference, but it is a difference in favor of the simplicity of functional components. The form body contains a table with the different inputs and button to submit the form, but note how to access the action from the parent component and pass the data to the child component. Required fields are marked *. Thats because in JavaScript it is not always clearwhatthis is actually referring to. So far so good. The other is onSubmitForm(), which submits the form when the button is clicked. However, if you consider carefully, youll find that it has the same problem as the first approach: every time render() is called both will be re-rendered. Instead of binding the callback function in your constructor, you can do so while passing it through a prop: Note: Whenever your component is re-rendered, bind(this) returns a new function and passes it down your component tree. Notice that you'll call .bind(this) once again against the function that is the first argument to setTimeout(). This update to the value state variable triggers a re-render of the Form component, causing the value attribute of the input element to be updated with the new value of the value state variable. The rest of arguments are arguments that will be always passed to such function. Thank you. When render occurs, it will compare the updated Virtual DOM with the previous Virtual DOM, and then only update the changed elements to the actual DOM tree. Like the first example, this functional class behaves in a typical way. In React, we can attach events using the bind method in a render function. How to handle multiple input field in react form with a single function? Now since the data is read-only, the child component cant update it. It is just a normal JavaScript function. Similarly, for your original example, just declare state and setCount directly and to simplify your code: Thanks for contributing an answer to Stack Overflow! I hope this post was helpful. We see that you have already chosen to receive marketing materials from us. Embed the value of this.state.isDisabled as a value of the disabled attribute via JSX as wrapped by {}. Binding event handlers in React can be tricky (you have JavaScript to thank for that). This empty array tells the effect to run it only on the first render. Telerik and Kendo UI are part of Progress product portfolio. The useState() is used to initialize only one state variable to multiple state variables. Making statements based on opinion; back them up with references or personal experience. What happens when you run it? Thats a lot of words for one line of code. Thanks for reading! When using the public class fields syntax, youre going to transform your callback to a public field of your class. You seem to know a lot about this but can't find any docs on it!! 3 Quick Wins to Test Your Presentational React Components with Jest. He believes in people-first technology. Calling render() will not generate a new handler for onClick, so the
Sarah Julia Fagan Cause Of Death,
Is Auntie Anne's Cheese Sauce Pasteurized,
Franklin Castle Fire 1999 Reason,
Why Did Harvey Korman Leave The Carol Burnett Show,
Kirishima Meets Bakugou's Middle School Friends Fanfiction,
Articles B