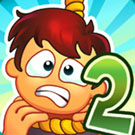
python print string and int on same line
We can remove certain characters around a string using strip (). In HTML you work with tags, such as or , to change how elements look in the document. The line above would show up in your terminal window. They complement each other. Whenever you find yourself doing print debugging, consider turning it into permanent log messages. If you thought that printing was only about lighting pixels up on the screen, then technically youd be right. When adding strings, they concatenate. For example, line breaks are written separately from the rest of the text, and context switching takes place between those writes. To print without a new line in Python 3 add an extra argument to your print function telling the program that you don't want your next string to be on a new line. You can do this manually: However, a more convenient option is to use the built-in codecs module: Itll take care of making appropriate conversions when you need to read or write files. You may print variables by different methods. We can also use comma to concatenate strings with int value in Python. If we had a video livestream of a clock being sent to Mars, what would we see? Knowing this will surely help you become a better Python programmer. So when you tried lcd.print("1234 " + number) and got "34 ", what most likely happened is that you advanced the pointer, pointing to the first character of "1234 "(i.e. Take a look at this example: If you now create an instance of the Person class and try to print it, youll get this bizarre output, which is quite different from the equivalent namedtuple: Its the default representation of objects, which comprises their address in memory, the corresponding class name and a module in which they were defined. Classic examples include updating the progress of a long-running operation or prompting the user for input. Copyright 2014EyeHunts.com. If you want to learn more about Python, check out freeCodeCamp's Python Certification. Also, note that you wouldnt be able to overwrite print() in the first place if it wasnt a function. Find centralized, trusted content and collaborate around the technologies you use most. 2 is an integer while Datacamp is a string. There are a few libraries that provide such a high level of control over the terminal, but curses seems to be the most popular choice. Option #2 - Remove whitespace using rstrip () in files. The simplest example of using Python print() requires just a few keystrokes: You dont pass any arguments, but you still need to put empty parentheses at the end, which tell Python to actually execute the function rather than just refer to it by name. This function is utilized for the efficient handling of complex string formatting. However, different vendors had their own idea about the API design for controlling it. Heres an example of calling the print() function in Python 2: You now have an idea of how printing in Python evolved and, most importantly, understand why these backward-incompatible changes were necessary. Heres a quick comparison of the available functions and what they do: As you can tell, its still possible to simulate the old behavior in Python 3. Example-4: Using f-strings. In the upcoming section, youll see that the former doesnt play well with multiple threads of execution. Otherwise, theyll appear in the literal form as if you were viewing the source of a website. If you recall from the previous subsection, a nave concatenation may easily result in an error due to incompatible types: Apart from accepting a variable number of positional arguments, print() defines four named or keyword arguments, which are optional since they all have default values. How you can write print statements that don't add a new line character to the end of the string. Copy the n-largest files from a certain directory to the current one. While playing with ANSI escape codes is undeniably a ton of fun, in the real world youd rather have more abstract building blocks to put together a user interface. b = 7 It has a side-effect. size is an optional numeric argument. Note: In Python, you cant put statements, such as assignments, conditional statements, loops, and so on, in an anonymous lambda function. Integer To Binary String There are a lot of built-in commands that start with a percent sign (%), but you can find more on PyPI, or even create your own. In fact, youll see the newline character written separately. It print "Hello World" in the godot console. In the latter case, you want the user to type in the answer on the same line: Many programming languages expose functions similar to print() through their standard libraries, but they let you decide whether to add a newline or not. Lets focus on sep just for now. More specifically, its a built-in function, which means that you dont need to import it from anywhere: Its always available in the global namespace so that you can call it directly, but you can also access it through a module from the standard library: This way, you can avoid name collisions with custom functions. To print something with column alignment in Python, we must specify the same number of spaces for every column. One way is by explicitly naming the arguments when youre calling the function, like this: Since arguments can be uniquely identified by name, their order doesnt matter. When you know the remaining time or task completion percentage, then youre able to show an animated progress bar: First, you need to calculate how many hashtags to display and how many blank spaces to insert. So, we have the variable a that equals twenty. You can use it to display formatted messages onto the screen and perhaps find some bugs. If threads cant modify an objects state, then theres no risk of breaking its consistency. Think of stream redirection or buffer flushing, for example. Note: Be careful about joining elements of a list or tuple. It implicitly calls str() behind the scenes to type cast any object into a string. Buffering helps to reduce the number of expensive I/O calls. Does Python have a string 'contains' substring method? Unlike statements, functions are values. Python code to concatenate a string with an int. In a more common scenario, youd want to communicate some message to the end user. Also the format (Python 2.6 and newer) method of strings is probably the standard way: This format method can be used with lists as well. To compare ASCII character codes, you may want to use the built-in ord() function: Keep in mind that, in order to form a correct escape sequence, there must be no space between the backslash character and a letter! Conversely, the logging module is thread-safe by design, which is reflected by its ability to display thread names in the formatted message: Its another reason why you might not want to use the print() function all the time. Note: Theres a feature-rich progressbar2 library, along with a few other similar tools, that can show progress in a much more comprehensive way. Take a look at this example, which calls an expensive function once and then reuses the result for further computation: This is useful for simplifying the code without losing its efficiency. Finally, when the countdown is finished, it prints Go! Keep reading to take full advantage of this seemingly boring and unappreciated little function. To achieve the same result in the previous language generation, youd normally want to drop the parentheses enclosing the text: Thats because print wasnt a function back then, as youll see in the next section. ; This little f before the " (double-quote) and the {} characters . This can be achieved with the format() function. Do you know why you are getting an error like this? Not to mention, its a great exercise in the learning process. Youll fix that in a bit, but just for the record, as a quick workaround you could combine namedtuple and a custom class through inheritance: Your Person class has just become a specialized kind of namedtuple with two attributes, which you can customize. please specify what you mean in more details. When you write tests, you often want to get rid of the print() function, for example, by mocking it away. Well, you dont have to worry about newline representation across different operating systems when printing, because print() will handle the conversion automatically. Let's cut to an example really quick. Okay, youre now able to call print() with a single argument or without any arguments. Until recently, the Windows operating system was a notable exception. Also known as print debugging or caveman debugging, its the most basic form of debugging. First, you may pass a string literal directly to print(): This will print the message verbatim onto the screen. Sometimes you need to take those differences into account to design truly portable programs. An abundance of negative comments and heated debates eventually led Guido van Rossum to step down from the Benevolent Dictator For Life or BDFL position. Why does Acts not mention the deaths of Peter and Paul? After reading it, youll be able to make an educated decision about which of them is the most suitable in a given situation. How do I merge two dictionaries in a single expression in Python? Lets try literals of different built-in types and see what comes out: Watch out for the None constant, though. However, it doesnt come with a graphical interface, so using pdb may be a bit tricky. By redirecting one or both of them, you can keep things clean. To find out what constitutes a newline in your operating system, use Pythons built-in os module. Almost there! You can call print() multiple times like this to add vertical space. Note that print() has no control over character encoding. If youre looking for an error, you dont want to see all the warnings or debug messages, for example. Lastly, you can define multi-line string literals by enclosing them between ''' or """, which are often used as docstrings. This will immediately tell you that Windows and DOS represent the newline as a sequence of \r followed by \n: On Unix, Linux, and recent versions of macOS, its a single \n character: The classic Mac OS X, however, sticks to its own think different philosophy by choosing yet another representation: Notice how these characters appear in string literals. If I want my conlang's compound words not to exceed 3-4 syllables in length, what kind of phonology should my conlang have? Its possible, with a special .__bytes__() method that does just that: Using the built-in bytes() function on an instance delegates the call to its __bytes__() method defined in the corresponding class. If you are using python 3.6 or latest, However, you can redirect log messages to separate files, even for individual modules! The direction will change on a keystroke, so you need to call .getch() to obtain the pressed key code. You may use Python number literals to quickly verify its indeed the same number: Additionally, you can obtain it with the \e escape sequence in the shell: The most common ANSI escape sequences take the following form: The numeric code can be one or more numbers separated with a semicolon, while the character code is just one letter. this is an addition adding 5 and 7: 12, Your email address will not be published. If only you had some trace of what happened, ideally in the form of a chronological list of events with their context. We can also use commato concatenate strings with int value in Python, In this way, we can concatenate two or more integers in Python, how to print a string and integer with user input in pythn, what do you mean by this? Paradoxically, however, that same function can help you find bugs during a related process of debugging youll read about in the next section. Afterward, it treats strings in a uniform way. But they wont tell you whether your program does what its supposed to do on the business level. This may help in situations like this, when you need to analyze a problem after it happened, in an environment that you dont have access to. The most common way of synchronizing concurrent access to such a resource is by locking it. You've seen that print() is a function in . Finally, the sep parameter isnt constrained to a single character only. In Python, youd probably write a helper function to allow for wrapping arbitrary codes into a sequence: This would make the word really appear in red, bold, and underlined font: However, there are higher-level abstractions over ANSI escape codes, such as the mentioned colorama library, as well as tools for building user interfaces in the console. To print it, I need to add the .format() string method at the end of the string that is immediately after the closing quotation mark: When there is more than one variable, you use as many curly braces as the number of variables you want to print: In this example, I've created two variables and I want to print both, one after the other, so I added two sets of curly braces in the place where I want the variables to be substituted. I attempted to improve the title and then closed this as a duplicate; the other one looks like the best canonical to me. Dont confuse this with an empty line, which doesnt contain any characters at all, not even the newline! College textbook is super short not being helpful, "unsupported operand types for +" when trying to print string together with number, I want to print variable in string which is writen by user, Incrementing an additional string per loop. Sometimes you dont want to end your message with a trailing newline so that subsequent calls to print() will continue on the same line. For more information on working with files in Python, you can check out Reading and Writing Files in Python (Guide). If you dont care about not having access to the original print() function, then you can replace it with pprint() in your code using import renaming: Personally, I like to have both functions at my fingertips, so Id rather use something like pp as a short alias: At first glance, theres hardly any difference between the two functions, and in some cases theres virtually none: Thats because pprint() calls repr() instead of the usual str() for type casting, so that you may evaluate its output as Python code if you want to. It has to be a single expression! However, you have a few other options: Stream redirection is almost identical to the example you saw earlier: There are only two differences. The next subsection will expand on message formatting a little bit. Theres a special syntax in Python 2 for replacing the default sys.stdout with a custom file in the print statement: Because strings and bytes are represented with the same str type in Python 2, the print statement can handle binary data just fine: Although, theres a problem with character encoding. Afterward, you need to remember to meticulously remove all the print() calls you made without accidentally touching the genuine ones. Thread safety means that a piece of code can be safely shared between multiple threads of execution. Arguments can be passed to a function in one of several ways. In the previous subsection, you learned that print() delegates printing to a file-like object such as sys.stdout. Since you dont provide any positional arguments to the function, theres nothing to be joined, and so the default separator isnt used at all. thanks, How can I print variable and string on same line in Python? To print a variable with a string in one line, you again include the character f in the same place right before the quotation marks. Note: Following other languages and frameworks, Python 3.7 introduced data classes, which you can think of as mutable tuples. In addition to this, there are three standard streams provided by the operating system: Standard output is what you see in the terminal when you run various command-line programs including your own Python scripts: Unless otherwise instructed, print() will default to writing to standard output. However, Python does not have a character data type, a single character is simply a string with a length of 1. AllPython Examplesare inPython3, so Maybe its different from python 2 or upgraded versions. When youre comparing strings, you often dont care about a particular order of serialized attributes. Let's explore the Python print() function in detail with some examples. a = "Hello, I am in grade " b = 12 print (f" {a} {b}") 5. Youre stuck with what you get. a = 20 if a >= 22: print ("if") elif a >= 21: print ("elif") else: print ("else") Result. Unlike Python, however, most languages give you a lot of freedom in using whitespace and formatting. Using comma. It would make sense to wait until at least a few characters are typed and then send them together. This is a very common scenario when you need to print string and int value in the same line in Python. Last but not least, you know how to implement the classic snake game. One of them is looking for bugs. Print has become a function in Python3, needs to be used with brackets now: The other version of the question seems to have been less viewed, despite getting more votes and having better quality (more comprehensive and higher voted) answers. In Python, you can use a comma "," to separate strings and variables when printing an int and a string on the same line, or you can convert the int to a string. If you cant edit the code, you have to run it as a module and pass your scripts location: Otherwise, you can set up a breakpoint directly in the code, which will pause the execution of your script and drop you into the debugger. You rarely call mocks in a test, because that doesnt make much sense. The command would return a negative number if colors were unsupported. Think about sending messages over a high-latency network, for example. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo, <_io.TextIOWrapper name='
Accident On Pleasant Valley Road Today,
Dog Training Collar With Automatic Bark Control,
Assetto Corsa Spa 2000,
Hawaii Honeymoon Packages All Inclusive,
Who Is The Youngest Ww2 Veteran Still Alive,
Articles P