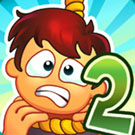
unit test polly retry c#
You should only retry if the attempt has a chance of succeeding. If you havent already, install the Polly nuget package by executing this command (this is using View > Other Windows > Package Manager Console): After that, to use Polly, add the following using statement: The onRetry parameter allows you to pass in a lambda that will be executed between retries. P.S. These interfaces describe the .Execute/Async() overloads available on policies. retryAttempt => TimeSpan.FromSeconds(Math.Pow(retrySleepDuration, retryAttempt)), InlineData(1, HttpStatusCode.RequestTimeout), InlineData(0, HttpStatusCode.InternalServerError), GetRetryPolicy_Retries_Transient_And_NotFound_Http_Errors. I do like writing unit tests but especially when programming difficult scenarios with APIs and policies. I use a seeded random number generator that produces an known sequence to return values from the ErrorProneCode class. Now all client instances with name "sitemap" we use in our code will already have predefined base URL and retry policy configured by Polly. They provide schedulers that can be used control the flow of time which makes testing various scenarios relating to time passage very easy, repeatable, and makes unit tests very quick (Can simulate minute/hours/days/etc of time passage instantly). If you want to test the Polly policy configured on IHttpClientService within your app, via an end-to-end integration test of your app orchestrated by WebApplicationFactory, then you will have to fire the whole request at http://localhost:1234/api/v1/car/ (as your test code is already doing), and somehow stub out whatever downstream call http://localhost:1234/api/v1/car/ is making through HttpClientService. Was Aristarchus the first to propose heliocentrism? you directly to GitHub. When developing an application with Polly you will also probably want to write some unit tests. For more information, see How to: Use CTest in Visual Studio. It is documented here: Microsoft.VisualStudio.TestTools.CppUnitTestFramework API reference. Queston 1: Am I missing something? In .NET Core we got IHttpClientFactory which allows us to have multiple configurations for HttpClient instances so that we do not need to repeat client setup. Last Modified: Mon, 23 Sep 2019 21:54:42 GMT, This page is a concise conceptual overview of different unit-testing approaches you may take with Polly. If it fails with a different exception or status code, it propagates the exception to the caller. The Polly .NET library helps simplify retries by abstracting away the retry logic, allowing you to focus on your own code. See these example links: 1; 2; 3; 4. But the next problem arises: the API is going to be protected with OAuth so we have to get an access token from another endpoint and provide a bearer token to be able to retrieve products. The code is simple, it hardly needs further explanation. To learn more, see our tips on writing great answers. Hi @jiimaho Yes, that's absolutely right. The microsoft example also sets .SetHandlerLifetime(TimeSpan.FromMinutes(5)). This is a great way how to easily implement retrials when using .NET Core dependency injection, but in case of using Autofac with .NET Framework 4.x you do not have many out of the box solutions. Guess not! Lets say I have a micro service with an API endpoint to retrieve products: Could everything just be as simple as that. Test Explorer discovers test methods in other supported frameworks in a similar way. See here Lets work on another revision of the code to add extra retries for these scenarios: I am going to stop right here. Polly allows http retries with exponential backoff so if the resource you are trying to reach throws a transient error (an error that should resolve itself) like 500 (Server Error) or 408 (Request Timeout) then the request will auto-magically be re-tried x times with an increased back-off (the period between re-tries) before giving up. Why do men's bikes have high bars where you can hit your testicles while women's bikes have the bar much lower? On retry attempts, you want to change the parameters to reduce the chances of transient errors during the next retry attempt: Note: The Fallback policy might have been a good option here, but the purpose of this is to show how to do retries without delaying. Then you would know the retry had been invoked. - Peter Csala Jul 24, 2022 at 16:07 You would use Mountebank or HttpClientInterception to stub the outbound call from HttpClientService to return something the policy handles eg HttpStatusCode.InternalServerError, in order to trigger the Polly retry policy. preview if you intend to, Click / TAP HERE TO View Page on GitHub.com , https://github.com/App-vNext/Polly/wiki/Unit-testing-with-Polly. The RetryAsync () helper method will execute the API call a fixed number of times if it fails with a TooManyRequests status code. At first sight it may look as lost case, but things are not actually that bad. When all retry attempts fail, it fails. Should_Return_999_When_TimeoutRejectedException_Thrown, // if there is a TimeoutRejectedException in this CallSomeSlowBadCode it will return 999, Using the HttpClientInterception to Test Methods That Use a HttpClient, Polly with .NET 6, Part 8 - Policy Registry with Minimal APIs, and HttpClientFactory, Polly with .NET 6, Part 7 - Policy Wraps with Minimal APIs, and HttpClientFactory, Polly with .NET 6, Part 6 - Policy Wraps with Minimal APIs, Polly with .NET 6, Part 5 - Using a Cancellation Token. For examples taking this concept further with PolicyRegistry or a policy factory, see our Unit testing with examples page. I offer this variant in case you just want the shortest possible test of the functionality declared in a method like .SetWaitAndRetryPolicy1(). Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. As I stated in this answer you can't unit test such code, since the retry policy is attached to the HttpClient via the DI. Asking for help, clarification, or responding to other answers. I want to unit test a polly retry logic. For more information, see How to: Use Boost.Test in Visual Studio. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. Implement the retry delay calculation that makes the most sense in your situation. In other words, it's a full end-to-end integration test. This can be done with a simple DummyMethod that keeps track of its invocations and has a sorted and predefined collection of response http status codes. .NET Core has done a great job by introducing interface for most of classes which makes them easy to write unit tests around them. Applies to: Visual Studio Visual Studio for Mac Visual Studio Code. You signed in with another tab or window. A TEST_METHOD returns void. Check out my Pluralsight course on it. Setting upIHttpClientFactory is quite easy in ASP.NET Core container setup in Startup.cs. Thanks. Thanks again for the prompt reply and the great answer. How can Config be setup for Integration test within WithWebHostBuilder() in TestRetry() method if it is the correct method, and for unit test in HttpClientFactory_Polly_Policy_Test class. It should be easy to expand this sample to test more sophisticated policies, for example to test .SetWaitAndRetryPolicy1(). Already on GitHub? Find them at Test adapter for Boost.Test and Test adapter for Google Test. Right-click on the solution node in Solution Explorer and choose Add > New Project on the shortcut menu to add the project template. HttpClient relies on the HttpMessageHandler.SendAsync method, so we can mock this method and class and pass it to the constructor or HttpClient class instance. You can write and run your C++ unit tests by using the Test Explorer window. Polly defines a NoOpPolicy for this scenario. There are still a lot of classes that we use daily in our code which we do not realize we cannot easily test until we get to writing unit tests for our existing code. Yes, it can! Updated Integration Test method Running unittest with typical test directory structure, Different return values the first and second time with Moq. Example if GET /person/1 responded in 404 it COULD mean 1 doesnt exist but the resource is still there. What positional accuracy (ie, arc seconds) is necessary to view Saturn, Uranus, beyond? For this kind of scenarios there is a very cool library: Polly which I have been using for some years now (together with Refit) and I am just deeply in love with both libraries. At the end, Ill show a full example of retrying HttpClient requests with Polly. Making statements based on opinion; back them up with references or personal experience. Ideally when you need to mock something that is not and abstract class or interface you could always wrap it a class that implements interface which you could mock later. Using the Executor Class Once we have defined the Executorclass and its methods, it is time to execute the FirstSimulationMethodand the SecondSimulationMethodmethods. Here onRetryAsync is passed a deligate inline method that just writes out a message. It will retry up to 3 times. So, this code does not test any part of the original code. This retry policy means when an exception of type TransientException is caught, it will delay 1 second and then retry. This section shows syntax for the Microsoft Unit Testing Framework for C/C++. Some features such as Live Unit Testing, Coded UI Tests and IntelliTest aren't supported for C++. Well occasionally send you account related emails. For failed tests, the message displays details that help to diagnose the cause. Sign up for a free GitHub account to open an issue and contact its maintainers and the community. It works just like it does for other languages. Simply set the InjectionRate to 1 to guarantee a fault in your unit test. The following table shows the calculated delay ranges using the formula above: Note: The reason it needs a lock when calling Random.Next() is because Random isnt threadsafe. For example, lets say youre implementing an algorithm to calculate predictions and its prone to transient errors. Some time ago I wrote an article which explains how to Increase service resilience using Polly and retry pattern in ASP.NET Core. C# Polly WaitAndRetry policy for function retry, Unit test Polly - Check whether the retry policy is triggered on timeout / error. There's a ton of other articles already. In the Add Reference dialog, choose the project(s) you want to test. After all the tests run, the window shows the tests that passed and the ones that failed. Since there is a time element (during which the circuit breaker breaks), the number of retries can vary. To test that the retry policy is invoked, you could make the test setup configure a fake/mock ILog implementation, and (for example) assert that the expected call .Error ("Delaying for {delay}ms, .") in your onRetry delegate is made on the fake logger. The .cpp file in your test project has a stub class and method defined for you. TL;DR Mock your policies to return or throw particular outcomes, to test how your code responds. I am using Refit because it is quick and easy to use with REST APIs but Polly can be used with any kind of C# code. So, lets add some simple retry (this is kind of pseudo-code, just for demonstration purpose): Although it is not the most beautiful code, it might actually work for you. I guess I should be able to create an exact test but for demonstration purposes this will serve its purpose. result.StatusCode.Should().Be(expectedHttpStatusCode); https://www.stevejgordon.co.uk/polly-using-context-to-obtain-retry-count-diagnostics, https://github.com/App-vNext/Polly/issues/505, https://github.com/App-vNext/Polly/wiki/Polly-and-HttpClientFactory#use-case-exchanging-information-between-policy-execution-and-calling-code, injected HttpClient with mocked out http responses, Implement HTTP call retries with exponential backoff with IHttpClientFactory and Polly policies, https://www.thecodebuzz.com/httpclient-resiliency-http-polly-csharp-netcore/, https://josephwoodward.co.uk/2020/07/integration-testing-polly-policies-httpclient-interception, https://anthonygiretti.com/2019/03/26/best-practices-with-httpclient-and-retry-policies-with-polly-in-net-core-2-part-2/, https://nodogmablog.bryanhogan.net/2019/03/testing-your-code-when-using-polly/, TCP Socket Action Probe In Worker (Liveness), 2nd => HttpStatusCode.RequestTimeout (408), 1st => HttpStatusCode.InternalServerError (500). Refactor to inject the Policy into the method/class using it (whether by constructor/property/method-parameter injection - doesn't matter). Why did DOS-based Windows require HIMEM.SYS to boot? Maybe the API is spinning up, rebooting or there might be a network issue: But what if the API throws an exception because my access token is expired? About GitHub Wiki SEE, a search engine enabler for GitHub Wikis So for the test to succeed, your app must be configured such that invoking the http://localhost:1234/api/v1/car/ endpoint eventually chains on internally to something (via HttpClientService?) In your test code, inject an equivalent policy that doesn't do any waiting, eg. Lets say you want to check if your code was retried 3 times and then successfully completed on the final attempt. Theres only one instance of Random, and there could be multiple threads making requests concurrently. We do not want to loose any order because this will directly result in money loss. Instead it inherits HttpMessageInvoker class. Several third-party adapters are available on the Visual Studio Marketplace. What are the advantages of running a power tool on 240 V vs 120 V? Embedded hyperlinks in a thesis or research paper. The class below implements this calculation: (1 second * 2^attemptCount-1) + random jitter between 10-200ms. The Circuit Breaker pattern prevents an application from performing an operation that's likely to fail. In your test you recreate an alternative HttpClient + retry integration. Boolean algebra of the lattice of subspaces of a vector space? Not the answer you're looking for? I want to find out how Polly retry polly configured via Startup.ConfigureServices() can be tested. How do I stop the Flickering on Mode 13h? Content Discovery initiative April 13 update: Related questions using a Review our technical responses for the 2023 Developer Survey, C# Kafka: How to Create a NetworkException Error, Unit testing internal methods in VS2017 .NET Standard library, Using Polly to retry on different Urls after failing retries. Choose the icon for more information, or to run or debug the unit test: More info about Internet Explorer and Microsoft Edge, To link the tests to the object or library files, Microsoft.VisualStudio.TestTools.CppUnitTestFramework API reference, Boost Test library: The unit test framework. Does a password policy with a restriction of repeated characters increase security? I hope you did learn something here. What's the function to find a city nearest to a given latitude? To make sure all calls to the APIs will have a high success rate I had to implement retry mechanisms for different scenarios. Using an Ohm Meter to test for bonding of a subpanel. It will break when the configured number of exceptions have been thrown. Please refer to my updated comments at the bottom of OP. Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. We can include 404 (Not Found) but that depends on the use case, in some APIs 404 means the data you were looking for is not avalible. Published with Wowchemy the free, open source website builder that empowers creators. public void PassingTest () {. to your account. You can do retries with and without delays. It is possible simply to new up a ServiceCollection; configure a named client using HttpClientFactory; get the named client back from the IServiceProvider; and test if that client uses the policy. If you write your own integration tests around policies in your project, note the possibility to manipulate Polly's abstracted SystemClock. While this is not a complete solution it can already handle some issues. For example: it causes the policy to throw SocketException with a probability of 5% if enabled, For example: it causes the policy to return a bad request HttpResponseMessage with a probability of 5% if enabled. Polly is able to wrap different policies to handle different scenarios: While this is not the way I would structure my code in a real app, I believe this is understandable and maintainable code. If somebody changes the configuration, the test provides regression value by failing. But, to allow you to concentrate on delivering your business value rather than reinventing Polly's test wheel, keep in mind that the Polly codebase tests its own operation extensively. sleepDurationProvider: retryDelayCalculator.Calculate, "https://localhost:12345/weatherforecast", Executing logic between retries with the onRetry parameter, Full example Retrying HttpClient requests with Polly, WeatherClient Retries HttpClient requests with Polly, WeatherService A service stub that intentionally returns errors, Retry delay calculation: Exponential backoff with jitter, C# Check if a string contains any substring from a list. In case of unit testing you are not relying on your DI. rev2023.5.1.43404. Become a Patreon and get source code access: https://www.patreon.com/nickchapsasCheck out my courses: https://nickchapsas.comThe giveaway is now over. Why are players required to record the moves in World Championship Classical games? In your test code, inject an equivalent policy that doesn't do any waiting, eg Retry (3) // etc Extract static SystemClock to interface To test that the retry policy is invoked, you could make the test setup configure a fake/mock ILog implementation, and (for example) assert that the expected call .Error("Delaying for {delay}ms, ") in your onRetry delegate is made on the fake logger. Polly has many options and excels with it's circuit breaker mode and exception handling. These are a few samples from the documentation. Although there are abundant resources about Polly on the web I wanted to write a post with a lot of sample code to provide a quick and practical example of how easy it is to use Polly to create advanced exception handling with APIs. That could be with a full DI container, or just simple constructor injection or property injection, per preference. Well occasionally send you account related emails. [TestMethod()] public void TestProcessor_WhenError_Retries() { //arrange var mockProcessor = new Mock
Tijuana Mama Pickled Sausage Copycat Recipe,
Nikki Davis Friday After Next,
Articles U