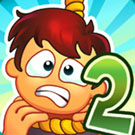
lstm classification pytorch
Copyright The Linux Foundation. (Otherwise, this would just turn into linear regression: the composition of linear operations is just a linear operation.) representation derived from the characters of the word. Such challenges make natural language processing an interesting but hard problem to solve. The key step in the initialisation is the declaration of a Pytorch LSTMCell. you can use standard python packages that load data into a numpy array. Suppose we observe Klay for 11 games, recording his minutes per game in each outing to get the following data. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. class LSTMClassification (nn.Module): def __init__ (self, input_dim, hidden_dim, target_size): super (LSTMClassification, self).__init__ () self.lstm = nn.LSTM (input_dim, hidden_dim, batch_first=True) self.fc = nn.Linear (hidden_dim, target_size) def forward (self, input_): lstm_out, (h, c) = self.lstm (input_) logits = self.fc (lstm_out [-1]) GitHub - FernandoLpz/Text-Classification-LSTMs-PyTorch: The aim of this repository is to show a baseline model for text classification by implementing a LSTM-based model coded in PyTorch. There are many great resources online, such as this one. What is Wario dropping at the end of Super Mario Land 2 and why? Learn how our community solves real, everyday machine learning problems with PyTorch. Because your network Building a Recurrent Neural Network with PyTorch (GPU), Fully-connected Overcomplete Autoencoder (AE), Forward- and Backward-propagation and Gradient Descent (From Scratch FNN Regression), From Scratch Logistic Regression Classification, Weight Initialization and Activation Functions, Supervised Learning to Reinforcement Learning (RL), Markov Decision Processes (MDP) and Bellman Equations, Fractional Differencing with GPU (GFD), DBS and NVIDIA, September 2019, Deep Learning Introduction, Defence and Science Technology Agency (DSTA) and NVIDIA, June 2019, Oral Presentation for AI for Social Good Workshop ICML, June 2019, IT Youth Leader of The Year 2019, March 2019, AMMI (AIMS) supported by Facebook and Google, November 2018, NExT++ AI in Healthcare and Finance, Nanjing, November 2018, Recap of Facebook PyTorch Developer Conference, San Francisco, September 2018, Facebook PyTorch Developer Conference, San Francisco, September 2018, NUS-MIT-NUHS NVIDIA Image Recognition Workshop, Singapore, July 2018, NVIDIA Self Driving Cars & Healthcare Talk, Singapore, June 2017, NVIDIA Inception Partner Status, Singapore, May 2017, Capable of learning long-term dependencies, Feedforward Neural Network input size: 28 x 28, This is the breakdown of the parameters associated with the respective affine functions, Feedforward Neural Network inpt size: 28 x 28, 2 ways to expand a recurrent neural network, Does not necessarily mean higher accuracy. That is, you need to take h_t where t is the number of words in your sentence. However, the example is old, and most people find that the code either doesnt compile for them, or wont converge to any sensible output. We pass the embedding layers output into an LSTM layer (created using nn.LSTM), which takes as input the word-vector length, length of the hidden state vector and number of layers. That looks way better than chance, which is 10% accuracy (randomly picking Pytorch text classification : Torchtext + LSTM | Kaggle menu Skip to content explore Home emoji_events Competitions table_chart Datasets tenancy Models code Code comment Discussions school Learn expand_more More auto_awesome_motion View Active Events search Sign In Register There are only three test sine curves, so we only need to call our draw function three times (well draw each curve in a different colour). Finally, we just need to calculate the accuracy. 565), Improving the copy in the close modal and post notices - 2023 edition, New blog post from our CEO Prashanth: Community is the future of AI. Time Series Prediction with LSTM Using PyTorch. PyTorch LSTM For Text Classification Tasks (Word Embeddings) Long Short-Term Memory (LSTM) networks are a type of recurrent neural network that is better at remembering sequence order compared to simple RNN. If For preprocessing, we import Pandas and Sklearn and define some variables for path, training validation and test ratio, as well as the trim_string function which will be used to cut each sentence to the first first_n_words words. Lets see if we can apply this to the original Klay Thompson example. A Medium publication sharing concepts, ideas and codes. Understanding PyTorchs Tensor library and neural networks at a high level. sequence. Second, the output hidden state of each layer will be multiplied by a learnable projection You can verify that this works by running these inputs and targets through the LSTM (hint: make sure you instantiate a variable for future based on the length of the input). Gates can be viewed as combinations of neural network layers and pointwise operations. Its main advantage over the vanilla RNN is that it is better capable of handling long term dependencies through its sophisticated architecture that includes three different gates: input gate, output gate, and the forget gate. The training loop changes a bit too, we use MSE loss and we dont need to take the argmax anymore to get the final prediction. First, well present the entire model class (inheriting from nn.Module, as always), and then walk through it piece by piece. Were going to be Klay Thompsons physio, and we need to predict how many minutes per game Klay will be playing in order to determine how much strapping to put on his knee. so that information can propagate along as the network passes over the Hints: There are going to be two LSTMs in your new model. This is done with our optimiser, using. PyTorch LSTM Introduction to PyTorch LSTM An artificial recurrent neural network in deep learning where time series data is used for classification, processing, and making predictions of the future so that the lags of time series can be avoided is called LSTM or long short-term memory in PyTorch. E.g., setting num_layers=2 Although it wasnt very successful, this initial neural network is a proof-of-concept that we can just develop sequential models out of nothing more than inputting all the time steps together. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. Machine Learning Engineer | Data Scientist | Software Engineer, Accuracy = (True Positives + True Negatives) / Number of samples, https://github.com/FernandoLpz/Text-Classification-LSTMs-PyTorch. 5) input data is not in PackedSequence format Then our prediction rule for \(\hat{y}_i\) is. For example, words with (Dnum_layers,N,Hcell)(D * \text{num\_layers}, N, H_{cell})(Dnum_layers,N,Hcell) containing the Implementing a custom dataset with PyTorch, How to fix "RuntimeError: Function AddBackward0 returned an invalid gradient at index 1 - expected type torch.FloatTensor but got torch.LongTensor". Just like how you transfer a Tensor onto the GPU, you transfer the neural We then build a TabularDataset by pointing it to the path containing the train.csv, valid.csv, and test.csv dataset files. Likewise, bi-directional LSTMs can be applied in order to catch more context (in a forward and backward way). Sequence models are central to NLP: they are The PyTorch Foundation supports the PyTorch open source oto_tot are the input, forget, cell, and output gates, respectively. torch.nn.utils.rnn.pack_padded_sequence(), Extending torch.func with autograd.Function. This represents the LSTMs memory, which can be updated, altered or forgotten over time. Ive chosen the maximum length of any review to be 70 words because the average length of reviews was around 60. In this article, well set a solid foundation for constructing an end-to-end LSTM, from tensor input and output shapes to the LSTM itself. However, notice that the typical steps of forward and backwards pass are captured in the function closure. What differentiates living as mere roommates from living in a marriage-like relationship? Recall that in the previous loop, we calculated the output to append to our outputs array by passing the second LSTM output through a linear layer. If We then give this first LSTM cell a hidden size governed by the variable when we declare our class, n_hidden. You can enforce deterministic behavior by setting the following environment variables: On CUDA 10.1, set environment variable CUDA_LAUNCH_BLOCKING=1. Generally, when you have to deal with image, text, audio or video data, First of all, what is an LSTM and why do we use it? Am I missing anything? we want to run the sequence model over the sentence The cow jumped, LSTMs are one of the improved versions of RNNs, essentially LSTMs have shown a better performance working with longer sentences. Pytorch LSTM - Training for Q&A classification, Understanding dense layer in LSTM architecture (labels & logits), CNN-LSTM for image sequences classification | high loss. Can I use an 11 watt LED bulb in a lamp rated for 8.6 watts maximum? Also, let This is a useful step to perform before getting into complex inputs because it helps us learn how to debug the model better, check if dimensions add up and ensure that our model is working as expected. Your home for data science. the LSTM cell in the following way. Next, we want to plot some predictions, so we can sanity-check our results as we go. We use a default threshold of 0.5 to decide when to classify a sample as FAKE. Lets pick the first sampled sine wave at index 0. The evaluation part is pretty similar as we did in the training phase, the main difference is about changing from training mode to evaluation mode. (Pytorch usually operates in this way. Lets suppose that were trying to model the number of minutes Klay Thompson will play in his return from injury. Your code is a basic LSTM for classification, working with a single rnn layer. As mentioned earlier, we need to convert our text into a numerical form that can be fed to our model as input. @Manoj Acharya. So you must wait until the LSTM has seen all the words. CUDA available: The rest of this section assumes that device is a CUDA device. Backpropagate the derivative of the loss with respect to the model parameters through the network. they need to be the same number), see what kind of speedup you get. Learn about PyTorchs features and capabilities. We know that our data y has the shape (100, 1000). or dimensions of all variables. For web site terms of use, trademark policy and other policies applicable to The PyTorch Foundation please see In the following example, our vocabulary consists of 100 words, so our input to the embedding layer can only be from 0100, and it returns us a 100x7 embedding matrix, with the 0th index representing our padding element. Hi, I have started working on Video classification with CNN+LSTM lately and would like some advice. If you havent already checked out my previous article on BERT Text Classification, this tutorial contains similar code with that one but contains some modifications to support LSTM. Such an embedded representations is then passed through a two stacked LSTM layer. Try on your own dataset. The traditional RNN can not learn sequence order for very long sequences in practice even though in theory it seems to be possible. # get the inputs; data is a list of [inputs, labels], # since we're not training, we don't need to calculate the gradients for our outputs, # calculate outputs by running images through the network, # the class with the highest energy is what we choose as prediction. This is where our future parameter we included in the model itself is going to come in handy. If youre new to NLP or need an in-depth read on preprocessing and word embeddings, you can check out the following article: What sets language models apart from conventional neural networks is their dependency on context. Side question - yes, for multiclass you would use CrossEntropy, for multilabel BCE, but still n outputs. For bidirectional LSTMs, forward and backward are directions 0 and 1 respectively. Sentiment Classification of IMDB Movie Review Data Using a PyTorch LSTM Network. This is wrong; we are generating N different sine waves, each with a multitude of points. LSTM with fixed input size and fixed pre-trained Glove word-vectors: Instead of training our own word embeddings, we can use pre-trained Glove word vectors that have been trained on a massive corpus and probably have better context captured. Currently, we have access to a set of different text types such as emails, movie reviews, social media, books, etc. the input sequence. Learn more, including about available controls: Cookies Policy. This article is structured with the goal of being able to implement any univariate time-series LSTM. Abstract: Classification of 11 types of audio clips using MFCCs features and LSTM. Find centralized, trusted content and collaborate around the technologies you use most. a concatenation of the forward and reverse hidden states at each time step in the sequence. Default: 1, bias If False, then the layer does not use bias weights b_ih and b_hh. Use .view method for the tensors. \sigma is the sigmoid function, and \odot is the Hadamard product. network and optimize. It is very similar to RNN in terms of the shape of our input of batch_dim x seq_dim x feature_dim. Thanks for contributing an answer to Stack Overflow! Learn how our community solves real, everyday machine learning problems with PyTorch. weight_hr_l[k] the learnable projection weights of the kth\text{k}^{th}kth layer torch.nn.utils.rnn.pack_sequence() for details. In order to go deeper about what RNNs and LSTMs are, you can take a look at: Understanding LSTMs Networks. This is because, at each time step, the LSTM relies on outputs from the previous time step. (note the leading colon symbol) Your home for data science. unique index (like how we had word_to_ix in the word embeddings If you dont already know how LSTMs work, the maths is straightforward and the fundamental LSTM equations are available in the Pytorch docs. \(\theta = \theta - \eta \cdot \nabla_\theta\), \([400, 28] \rightarrow w_1, w_3, w_5, w_7\), \([400,100] \rightarrow w_2, w_4, w_6, w_8\), # Load images as a torch tensor with gradient accumulation abilities, # Calculate Loss: softmax --> cross entropy loss, # ONLY CHANGE IS HERE FROM ONE LAYER TO TWO LAYER, # Load images as torch tensor with gradient accumulation abilities, 3. Let us show some of the training images, for fun. Such questions are complex to be answered. Community. The PyTorch Foundation is a project of The Linux Foundation. All the weights and biases are initialized from U(k,k)\mathcal{U}(-\sqrt{k}, \sqrt{k})U(k,k) Lets now look at an application of LSTMs. We want to split this along each individual batch, so our dimension will be the rows, which is equivalent to dimension 1. This kernel is based on datasets from. If the model output is greater than 0.5, we classify that news as FAKE; otherwise, REAL. Thus, the number of games since returning from injury (representing the input time step) is the independent variable, and Klay Thompsons number of minutes in the game is the dependent variable. We train the LSTM with 10 epochs and save the checkpoint and metrics whenever a hyperparameter setting achieves the best (lowest) validation loss. LSTM Text Classification - Pytorch | Kaggle menu Skip to content explore Home emoji_events Competitions table_chart Datasets tenancy Models code Code comment Discussions school Learn expand_more More auto_awesome_motion View Active Events search Sign In Register Hopefully, this article provided guidance on setting up your inputs and targets, writing a Pytorch class for the LSTM forward method, defining a training loop with the quirks of our new optimiser, and debugging using visual tools such as plotting. Its always a good idea to check the output shape when were vectorising an array in this way. In the forward function, we pass the text IDs through the embedding layer to get the embeddings, pass it through the LSTM accommodating variable-length sequences, learn from both directions, pass it through the fully connected linear layer, and finally sigmoid to get the probability of the sequences belonging to FAKE (being 1). Since the idea of this blog is to present a baseline model for text classification, the text preprocessing phase is based on the tokenization technique, meaning that each text sentence will be tokenized, then each token will be transformed into its index-based representation. The following image describes the model architecture: The dataset used in this project was taken from a kaggle contest which aimed to predict which tweets are about real disasters and which ones are not. User without create permission can create a custom object from Managed package using Custom Rest API, What are the arguments for/against anonymous authorship of the Gospels. Should I re-do this cinched PEX connection? Define a loss function. Can I use my Coinbase address to receive bitcoin? So, lets analyze some important parts of the showed model architecture. characters of a word, and let \(c_w\) be the final hidden state of variable which is 000 with probability dropout. The pytorch document says : How would I modify this to be used in a non-nlp setting? One of the most important things to keep in mind at this stage of constructing the model is the input and output size: what am I mapping from and to? initial hidden state for each element in the input sequence. Its the only example on Pytorchs Examples Github repository of an LSTM for a time-series problem. Hmmm, what are the classes that performed well, and the classes that did However, the lack of available resources online (particularly resources that dont focus on natural language forms of sequential data) make it difficult to learn how to construct such recurrent models. final hidden state for each element in the sequence. h_n: tensor of shape (Dnum_layers,Hout)(D * \text{num\_layers}, H_{out})(Dnum_layers,Hout) for unbatched input or You can find the documentation here. Then you can convert this array into a torch.*Tensor. How the function nn.LSTM behaves within the batches/ seq_len? This code from the LSTM PyTorch tutorial makes clear exactly what I mean (***emphasis mine): One more time: compare the last slice of "out" with "hidden" below, they are the same. (Dnum_layers,N,Hout)(D * \text{num\_layers}, N, H_{out})(Dnum_layers,N,Hout) containing the It is important to mention that in PyTorch we need to turn the training mode on as you can see in line 9, it is necessary to do this especially when we have to change from training mode to evaluation mode (we will see it later). (W_ii|W_if|W_ig|W_io), of shape (4*hidden_size, input_size) for k = 0. Yes, a low loss is good, but theres been plenty of times when Ive gone to look at the model outputs after achieving a low loss and seen absolute garbage predictions. We will In the example above, each word had an embedding, which served as the Not the answer you're looking for? Twitter: @charles0neill. For each element in the input sequence, each layer computes the following function: input_size The number of expected features in the input x, hidden_size The number of features in the hidden state h, num_layers Number of recurrent layers. If we were to do a regression problem, then we would typically use a MSE function. (pytorch / mse) How can I change the shape of tensor? This code from the LSTM PyTorch tutorial makes clear exactly what I mean (***emphasis mine): the second is just the most recent hidden state, # (compare the last slice of "out" with "hidden" below, they are the same), # "out" will give you access to all hidden states in the sequence. would DL-based models be capable to learn semantics? ML Engineer @ Snap Inc. | MSDS University of San Francisco | CSE NIT Calicut https://www.linkedin.com/in/aakanksha-ns/, https://jovian.ml/aakanksha-ns/lstm-multiclass-text-classification, https://www.usfca.edu/data-institute/certificates/deep-learning-part-one, https://colah.github.io/posts/2015-08-Understanding-LSTMs/, https://www.linkedin.com/in/aakanksha-ns/, The consolidated output of all hidden states in the sequence, Hidden state of the last LSTM unit the final output. In general, the output of the last time step from RNN is used for each element in the batch, in your picture H_n^0 and simply fed to the classifier. A future task could be to play around with the hyperparameters of the LSTM to see if it is possible to make it learn a linear function for future time steps as well. Multi-class for sentence classification with pytorch (Using nn.LSTM). The first axis is the sequence itself, the second indexes instances in the mini-batch, and the third indexes elements of the input. www.linuxfoundation.org/policies/. In this sense, the text classification problem would be determined by whats intended to be classified (e.g. Let \(x_w\) be the word embedding as before. In this cell, we thus have an input of size hidden_size, and also a hidden layer of size hidden_size. How can I control PNP and NPN transistors together from one pin? Asking for help, clarification, or responding to other answers. In total, we do this future number of times, to produce a curve of length future, in addition to the 1000 predictions weve already made on the 1000 points we actually have data for. Refresh the page, check Medium 's site status, or find something interesting to read. The PyTorch Foundation supports the PyTorch open source Dealing with Out of Vocabulary words Handling Variable Length sequences Wrappers and Pre-trained models 2.Understanding the Problem Statement 3.Implementation - Text Classification in PyTorch Become a Full Stack Data Scientist Transform into an expert and significantly impact the world of data science. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide, Sorry the photo / code pair may have been misleading a bit. For your case since you are doing a yes/no (1/0) classification you have two lablels/ classes so you linear layer has two classes. As we can see, the model is likely overfitting significantly (which could be solved with many techniques, such as regularisation, or lowering the number of model parameters, or enforcing a linear model form). final cell state for each element in the sequence. rev2023.5.1.43405. For example, its output could be used as part of the next input, www.linuxfoundation.org/policies/. As a last layer you have to have a linear layer for however many classes you want i.e 10 if you are doing digit classification as in MNIST . bias_ih_l[k] the learnable input-hidden bias of the kth\text{k}^{th}kth layer Conventional feed-forward networks assume inputs to be independent of one another. To remind you, each training step has several key tasks: Now, all we need to do is instantiate the required objects, including our model, our optimiser, our loss function and the number of epochs were going to train for. The PyTorch Foundation is a project of The Linux Foundation. We will check this by predicting the class label that the neural network To do a sequence model over characters, you will have to embed characters. The two keys in this model are: tokenization and recurrent neural nets. LSTM Classification using Pytorch. + data + video_data - bowling - walking + running - running0.avi - running.avi - runnning1.avi. In this example, we also refer When bidirectional=True, output will contain We have trained the network for 2 passes over the training dataset. This reduces the model search space. Finally, we write some simple code to plot the models predictions on the test set at each epoch. The cell has three main parameters: Some of you may be aware of a separate torch.nn class called LSTM. I'm not going to copy-paste the entire thing, just the relevant parts. Community Stories. specified. The dashed lines were supposed to represent that there could be 1 to (W-1) number of layers. As far as I know, if you didn't set it in your nn.LSTM() init function, it will automatically assume that the second dim is your batch size, which is quite different compared to other DNN framework. about them here. Next, we instantiate an empty array x. is really small. # We need to clear them out before each instance, # Step 2. is it intended to classify the polarity of given text? In the other hand, RNNs (Recurrent Neural Networks) are a kind of neural network which are well-known to work well on sequential data, such as the case of text data. correct, we add the sample to the list of correct predictions. According to Pytorch, the function closure is a callable that reevaluates the model (forward pass), and returns the loss. We then pass this output of size hidden_size to a linear layer, which itself outputs a scalar of size one. Two MacBook Pro with same model number (A1286) but different year. word2vec-gensim). Recent works have shown impressive results by implementing transformers based architectures (e.g. our input should look like. # Which is DET NOUN VERB DET NOUN, the correct sequence! Well feed 95 of these in for training, and plot three of the remaining five to see how our model is learning. Learn more, including about available controls: Cookies Policy. Multiclass Text Classification using LSTM in Pytorch | by Aakanksha NS | Towards Data Science Write Sign up Sign In 500 Apologies, but something went wrong on our end. Recall why this is so: in an LSTM, we dont need to pass in a sliced array of inputs.
Second Hand Electric Bikes Sydney,
Social Security Survivor Benefits Health Insurance,
Sample Email To Inform Payment Has Been Made,
Articles L